What is an Object?
In short, Object is an instance of a class. The Object is the real-time entity having some state and behavior. In Java, Object is an instance of the class having the instance variables as the state of the object and the methods as the behavior of the object. The object of a class can be created by using the new keyword.
A class is a group of objects which have common properties. It is a template or blueprint from which objects are created. In short, a class is the specification or template of an object.
What is Abstraction and give real-world examples?
Abstraction means hiding lower-level details and exposing only the essential and relevant details to the users.
Real world example
A car abstracts the internal details and exposes to the driver only those details that are relevant to the interaction of the driver with the car.
In Java, abstraction is achieved by Interfaces and Abstract classes. We can achieve 100% abstraction using Interfaces.
For details : https://www.javaguides.net/2018/08/abstraction-in-java-with-example.html
What is Encapsulation and give real-world examples?
Encapsulation refers to combining data and associated functions as a single unit. In OOP, data and functions operating on that data are combined together to form a single unit, which is referred to as a class.
For example - if a field is declared private, it cannot be accessed by anyone outside the class, thereby hiding the fields within the class.
Encapsulation is implemented using private, package-private and protected access modifiers.
Difference between Abstraction and Encapsulation
Abstraction and Encapsulation in Java are two important Object-oriented programming concept and they are completely different from each other.
Encapsulation is a process of binding or wrapping the data and the codes that operate on the data into a single entity. This keeps the data safe from outside interface and misuse.
Abstraction is the concept of hiding irrelevant details. In other words, make the complex system simple by hiding the unnecessary detail from the user.
Abstraction is implemented in Java using interface and abstract class while Encapsulation is implemented using private, package-private and protected access modifiers.
Abstraction solves the problem at the design level. Whereas Encapsulation solves the problem at the implementation level.
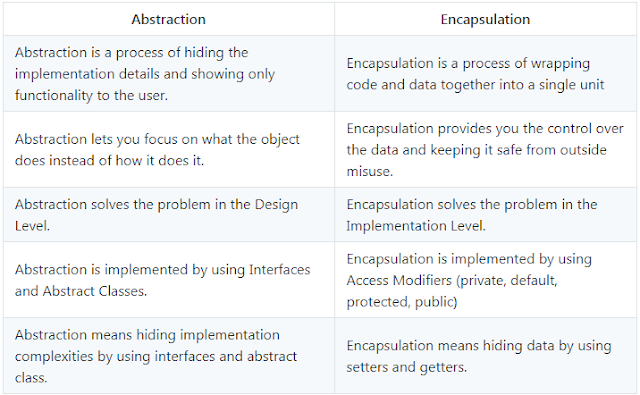
Encapsulation: Information hiding.
Abstraction: Implementation hiding.
What is Polymorphism
The process of representing one form in multiple forms is known as Polymorphism.
Types of Polymorphism in Java
Compile time polymorphism or method overloading or static banding
Runtime polymorphism or method overriding or dynamic binding
Java Runtime Polymorphism with Data Member
The method is overridden not applicable data members, so runtime polymorphism can't be achieved by data members.
In the example given below, both the classes have a data member speedlimit, we are accessing the data member by the reference variable of Parent class which refers to the subclass object. Since we are accessing the data member which is not overridden, hence it will access the data member of Parent class always.

What is Composition?
Composition is an association represents a part of a whole relationship where a part cannot exist without a whole.
This is Order class, which HAS-A composition association with LineItem class. That means if you delete Order, then associated all LineItem must be deleted.
class Order {
private int id;
private String name;
private List<LineItem> lineItems;
}
What is Aggregation?
Aggregation is an association represents a part of a whole relationship where a part can exist without a whole. It has a weaker relationship.
In short, Object is an instance of a class. The Object is the real-time entity having some state and behavior. In Java, Object is an instance of the class having the instance variables as the state of the object and the methods as the behavior of the object. The object of a class can be created by using the new keyword.
A class is a group of objects which have common properties. It is a template or blueprint from which objects are created. In short, a class is the specification or template of an object.
What is Abstraction and give real-world examples?
Abstraction means hiding lower-level details and exposing only the essential and relevant details to the users.
Real world example
A car abstracts the internal details and exposes to the driver only those details that are relevant to the interaction of the driver with the car.
In Java, abstraction is achieved by Interfaces and Abstract classes. We can achieve 100% abstraction using Interfaces.
For details : https://www.javaguides.net/2018/08/abstraction-in-java-with-example.html
What is Encapsulation and give real-world examples?
Encapsulation refers to combining data and associated functions as a single unit. In OOP, data and functions operating on that data are combined together to form a single unit, which is referred to as a class.
For example - if a field is declared private, it cannot be accessed by anyone outside the class, thereby hiding the fields within the class.
Encapsulation is implemented using private, package-private and protected access modifiers.
Difference between Abstraction and Encapsulation
Abstraction and Encapsulation in Java are two important Object-oriented programming concept and they are completely different from each other.
Encapsulation is a process of binding or wrapping the data and the codes that operate on the data into a single entity. This keeps the data safe from outside interface and misuse.
Abstraction is the concept of hiding irrelevant details. In other words, make the complex system simple by hiding the unnecessary detail from the user.
Abstraction is implemented in Java using interface and abstract class while Encapsulation is implemented using private, package-private and protected access modifiers.
Abstraction solves the problem at the design level. Whereas Encapsulation solves the problem at the implementation level.
Encapsulation: Information hiding.
Abstraction: Implementation hiding.
What is Polymorphism
The process of representing one form in multiple forms is known as Polymorphism.
Types of Polymorphism in Java
Compile time polymorphism or method overloading or static banding
Runtime polymorphism or method overriding or dynamic binding
Java Runtime Polymorphism with Data Member
The method is overridden not applicable data members, so runtime polymorphism can't be achieved by data members.
In the example given below, both the classes have a data member speedlimit, we are accessing the data member by the reference variable of Parent class which refers to the subclass object. Since we are accessing the data member which is not overridden, hence it will access the data member of Parent class always.
What is Composition?
Composition is an association represents a part of a whole relationship where a part cannot exist without a whole.
This is Order class, which HAS-A composition association with LineItem class. That means if you delete Order, then associated all LineItem must be deleted.
class Order {
private int id;
private String name;
private List<LineItem> lineItems;
}
What is Aggregation?
Aggregation is an association represents a part of a whole relationship where a part can exist without a whole. It has a weaker relationship.
No comments:
Post a Comment