Categories of Java Design patterns
Creational patterns
Factory method/Template
Abstract Factory
Builder
Prototype
Singleton
Structural patterns
Adapter
Bridge
Filter
Composite
Decorator
Facade
Flyweight
Proxy
Behavioral patterns
Interpreter
Template method/ pattern
Chain of responsibility
Command pattern
Iterator pattern
Strategy pattern
Visitor pattern
Observer design pattern
J2EE patterns
MVC Pattern
Data Access Object pattern
Front controller pattern
Intercepting filter pattern
Transfer object pattern
These design patterns are all about class instantiation or object creation. These patterns can be further categorized into Class-creational patterns and object-creational patterns. While class-creation patterns use inheritance effectively in the instantiation process, object-creation patterns use delegation effectively to get the job done.
Use case of creational design pattern-
1) Suppose a developer wants to create a simple DBConnection class to connect to a database and wants to access the database at multiple locations from code, generally what developer will do is create an instance of DBConnection class and use it for doing database operations wherever required. Which results in creating multiple connections from the database as each instance of DBConnection class will have a separate connection to the database. In order to deal with it, we create DBConnection class as a singleton class, so that only one instance of DBConnection is created and a single connection is established. Because we can manage DB Connection via one instance so we can control load balance, unnecessary connections, etc.
Use Case Of Structural Design Pattern-
These design patterns are about organizing different classes and objects to form larger structures and provide new functionality.
1) When 2 interfaces are not compatible with each other and want to make establish a relationship between them through an adapter its called adapter design pattern. Adapter pattern converts the interface of a class into another interface or classes the client expects that is adapter lets classes works together that could not otherwise because of incompatibility. so in these type of incompatible scenarios, we can go for the adapter pattern.
Behavioral patterns are about identifying common communication patterns between objects and realize these patterns.
Behavioral patterns are Chain of responsibility, Command, Interpreter, Iterator, Mediator, Memento, Null Object, Observer, State, Strategy, Template method, Visitor
Use Case of Behavioral Design Pattern-
1) Template pattern defines the skeleton of an algorithm in an operation deferring some steps to sub-classes, Template method lets subclasses redefine certain steps of an algorithm without changing the algorithm structure. say for an example in your project you want the behavior of the module can be extended, such that we can make the module behave in new and different ways as the requirements of the application change, or to meet the needs of new applications. However, No one is allowed to make source code changes to it. it means you can add but can’t modify the structure in those scenarios a developer can approach template design pattern.
2) Suppose you want to create multiple instances of similar kind and want to achieve loose coupling then you can go for Factory pattern. A class implementing factory design pattern works as a bridge between multiple classes. Consider an example of using multiple database servers like SQL Server and Oracle. If you are developing an application using SQL Server database as back end, but in future need to change database to oracle, you will need to modify all your code, so as factory design patterns maintain loose coupling and easy implementation we should go for factory for achieving loose coupling and creation of similar kind of object.
Factory Method :
Used to create loosely coupled objects.
Observer design pattern
The Observer Pattern defines a one to many dependency between objects so that one object changes state, all of its dependents are notified and updated automatically.
Suppose we are building a cricket app that notifies viewers about the information such as current score, run rate etc. Suppose we have made two display elements CurrentScoreDisplay and AverageScoreDisplay. CricketData has all the data (runs, bowls etc.) and whenever data changes the display elements are notified with new data and they display the latest data accordingly
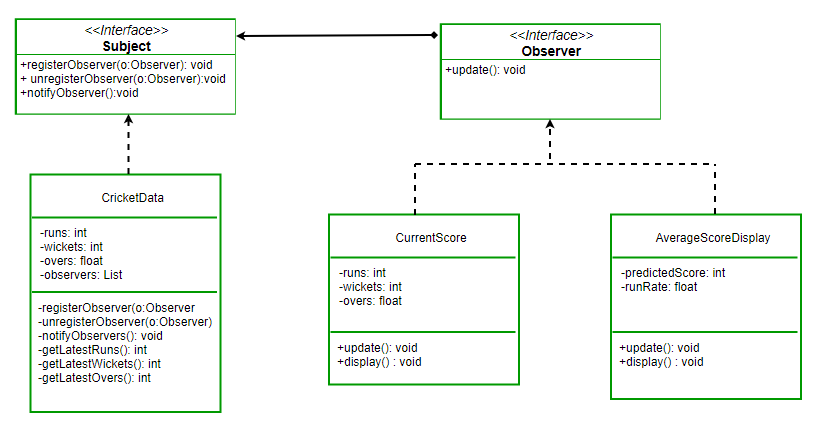
Implementation Link : https://www.geeksforgeeks.org/observer-pattern-set-2-implementation/
Singleton Design Pattern
To be used when only one instance of the class is required,
https://www.geeksforgeeks.org/singleton-design-pattern/
java.lang.Runtime and java.awt.Desktop are 2 singleton classes provided by JVM.
Application : Cache, ConfigurationFile, DB Classes
How to prevent Singleton Pattern from Reflection, Serialization and Cloning?
Reflection : Use Enum
As enums don’t have any constructor so it is not possible for Reflection to utilize it. Enums have their by-default constructor, we can’t invoke them by ourself. JVM handles the creation and invocation of enum constructors internally. As enums don’t give their constructor definition to the program, it is not possible for us to access them by Reflection also. Hence, reflection can’t break singleton property in case of enums.
Overcome serialization issue:- To overcome this issue, we have to implement method readResolve() method.
// implement readResolve method
protected Object readResolve()
{
return instance;
}
Overcome Cloning issue:- To overcome this issue, override clone() method and throw an exception from clone method that is CloneNotSupportedException.
Decorator Pattern
The decorator pattern attaches additional responsibilities to an object dynamically. Decorators provide a flexible alternative to subclassing for extending functionality.
The decorator pattern can be used to make it possible to extend (decorate) the functionality of a certain object at runtime.
The decorator pattern is an alternative to subclassing. Subclassing adds behavior at compile time, and the change affects all instances of the original class; decorating can provide new behavior at runtime for individual objects.
Decorator offers a pay-as-you-go approach to adding responsibilities. Instead of trying to support all foreseeable features in a complex, customizable class, you can define a simple class and add functionality incrementally with Decorator objects.
https://www.geeksforgeeks.org/decorator-pattern-set-3-coding-the-design/
When to use Strategy Design Pattern in Java?
https://www.geeksforgeeks.org/strategy-pattern-set-2/
One of a good example of Strategy pattern from JDK itself is a Collections.sort() method and Comparator interface, which is a strategy interface and defines a strategy for comparing objects. Because of this pattern, we don't need to modify sort() method (closed for modification) to compare any object, at the same time we can implement Comparator interface to define new comparing strategy (open for extension).
Facade Design Pattern
So, As the name suggests, it means the face of the building. The people walking past the road can only see this glass face of the building. They do not know anything about it, the wiring, the pipes and other complexities. It hides all the complexities of the building and displays a friendly face.
Example : JDBC getConnection();
https://www.geeksforgeeks.org/facade-design-pattern-introduction/
Chain of Responsibility Design Pattern
Chain of Responsibility allows a number of classes to attempt to handle a request, independently of any other object along the chain. Once the request is handled, it completes it’s journey through the chain.
javax.servlet.Filter#doFilter()
The doFilter method of the Filter is called by the container each time a request/response pair is passed through the chain due to a client request for a resource at the end of the chain. The FilterChain passed in to this method allows the Filter to pass on the request and response to the next entity in the chain.
java.util.logging.Logger#log
If the logger is currently enabled for the given message level then the given message is forwarded to all the registered output Handler objects.
Adapter Design Pattern in Java
Adapter design pattern is one of the structural design pattern and its used so that two unrelated interfaces can work together. The object that joins these unrelated interface is called an Adapter.
BufferedReader br = new BufferedReader(new InputStreamReader(System.in));
System.out.print("Enter String");
String s = br.readLine();
System.out.print("Enter input: " + s);
Now observe above code carefully.
1) System.in is static instance of InputStream declared as:
public final static InputStream in = nullInputStream();
This input stream reads the data from console in bytes stream.
2) BufferedReader as java docs define, reads a character stream.
//Reads text from a character-input stream, buffering characters so as to
//provide for the efficient reading of characters, arrays, and lines.
public class BufferedReader extends Reader{..}
Now here is the problem. System.in provides byte stream where BufferedReader expects character stream. How they will work together?
This is the ideal situation to put a adapter in between two incompatible interfaces. InputStreamReader does exactly this thing and works adapter between System.in and BufferedReader.
/** An InputStreamReader is a bridge from byte streams to character streams:
* It reads bytes and decodes them into characters using a specified charset.
* The charset that it uses may be specified by name or may be given explicitly,
* or the platform's default charset may be accepted.
*/
public class InputStreamReader extends Reader {...}
1) java.util.Arrays#asList()
This method accepts multiple strings and return a list of input strings. Though it’s very basic usage, but it’s what a adapter does, right?
2) java.io.OutputStreamWriter(OutputStream)
It’s similar to above usecase we discussed in this post:
Writer writer = new OutputStreamWriter(new FileOutputStream("c:\\data\\output.txt"));
writer.write("Hello World");
3) javax.xml.bind.annotation.adapters.XmlAdapter#marshal() and #unmarshal()
Adapts a Java type for custom marshaling. Convert a bound type to a value type.
Creational patterns
Factory method/Template
Abstract Factory
Builder
Prototype
Singleton
Structural patterns
Adapter
Bridge
Filter
Composite
Decorator
Facade
Flyweight
Proxy
Behavioral patterns
Interpreter
Template method/ pattern
Chain of responsibility
Command pattern
Iterator pattern
Strategy pattern
Visitor pattern
Observer design pattern
J2EE patterns
MVC Pattern
Data Access Object pattern
Front controller pattern
Intercepting filter pattern
Transfer object pattern
These design patterns are all about class instantiation or object creation. These patterns can be further categorized into Class-creational patterns and object-creational patterns. While class-creation patterns use inheritance effectively in the instantiation process, object-creation patterns use delegation effectively to get the job done.
Use case of creational design pattern-
1) Suppose a developer wants to create a simple DBConnection class to connect to a database and wants to access the database at multiple locations from code, generally what developer will do is create an instance of DBConnection class and use it for doing database operations wherever required. Which results in creating multiple connections from the database as each instance of DBConnection class will have a separate connection to the database. In order to deal with it, we create DBConnection class as a singleton class, so that only one instance of DBConnection is created and a single connection is established. Because we can manage DB Connection via one instance so we can control load balance, unnecessary connections, etc.
Use Case Of Structural Design Pattern-
These design patterns are about organizing different classes and objects to form larger structures and provide new functionality.
1) When 2 interfaces are not compatible with each other and want to make establish a relationship between them through an adapter its called adapter design pattern. Adapter pattern converts the interface of a class into another interface or classes the client expects that is adapter lets classes works together that could not otherwise because of incompatibility. so in these type of incompatible scenarios, we can go for the adapter pattern.
Behavioral patterns are about identifying common communication patterns between objects and realize these patterns.
Behavioral patterns are Chain of responsibility, Command, Interpreter, Iterator, Mediator, Memento, Null Object, Observer, State, Strategy, Template method, Visitor
Use Case of Behavioral Design Pattern-
1) Template pattern defines the skeleton of an algorithm in an operation deferring some steps to sub-classes, Template method lets subclasses redefine certain steps of an algorithm without changing the algorithm structure. say for an example in your project you want the behavior of the module can be extended, such that we can make the module behave in new and different ways as the requirements of the application change, or to meet the needs of new applications. However, No one is allowed to make source code changes to it. it means you can add but can’t modify the structure in those scenarios a developer can approach template design pattern.
2) Suppose you want to create multiple instances of similar kind and want to achieve loose coupling then you can go for Factory pattern. A class implementing factory design pattern works as a bridge between multiple classes. Consider an example of using multiple database servers like SQL Server and Oracle. If you are developing an application using SQL Server database as back end, but in future need to change database to oracle, you will need to modify all your code, so as factory design patterns maintain loose coupling and easy implementation we should go for factory for achieving loose coupling and creation of similar kind of object.
Factory Method :
Used to create loosely coupled objects.
Observer design pattern
The Observer Pattern defines a one to many dependency between objects so that one object changes state, all of its dependents are notified and updated automatically.
Suppose we are building a cricket app that notifies viewers about the information such as current score, run rate etc. Suppose we have made two display elements CurrentScoreDisplay and AverageScoreDisplay. CricketData has all the data (runs, bowls etc.) and whenever data changes the display elements are notified with new data and they display the latest data accordingly
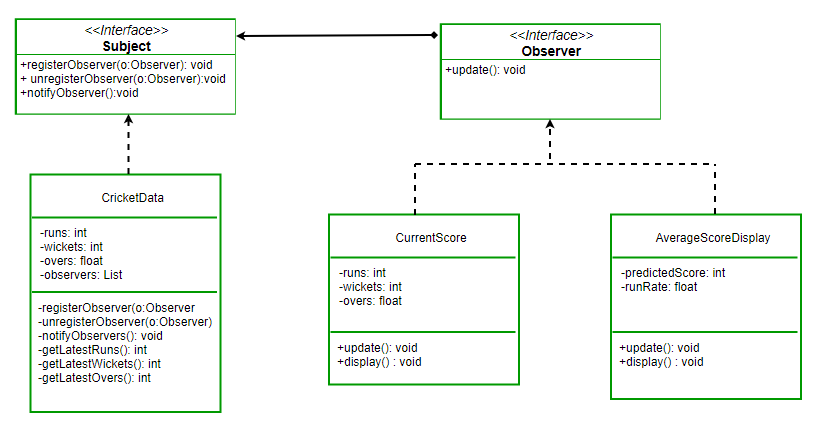
Implementation Link : https://www.geeksforgeeks.org/observer-pattern-set-2-implementation/
Singleton Design Pattern
To be used when only one instance of the class is required,
https://www.geeksforgeeks.org/singleton-design-pattern/
java.lang.Runtime and java.awt.Desktop are 2 singleton classes provided by JVM.
Application : Cache, ConfigurationFile, DB Classes
How to prevent Singleton Pattern from Reflection, Serialization and Cloning?
Reflection : Use Enum
As enums don’t have any constructor so it is not possible for Reflection to utilize it. Enums have their by-default constructor, we can’t invoke them by ourself. JVM handles the creation and invocation of enum constructors internally. As enums don’t give their constructor definition to the program, it is not possible for us to access them by Reflection also. Hence, reflection can’t break singleton property in case of enums.
Overcome serialization issue:- To overcome this issue, we have to implement method readResolve() method.
// implement readResolve method
protected Object readResolve()
{
return instance;
}
Overcome Cloning issue:- To overcome this issue, override clone() method and throw an exception from clone method that is CloneNotSupportedException.
Decorator Pattern
The decorator pattern attaches additional responsibilities to an object dynamically. Decorators provide a flexible alternative to subclassing for extending functionality.
The decorator pattern can be used to make it possible to extend (decorate) the functionality of a certain object at runtime.
The decorator pattern is an alternative to subclassing. Subclassing adds behavior at compile time, and the change affects all instances of the original class; decorating can provide new behavior at runtime for individual objects.
Decorator offers a pay-as-you-go approach to adding responsibilities. Instead of trying to support all foreseeable features in a complex, customizable class, you can define a simple class and add functionality incrementally with Decorator objects.
https://www.geeksforgeeks.org/decorator-pattern-set-3-coding-the-design/
When to use Strategy Design Pattern in Java?
https://www.geeksforgeeks.org/strategy-pattern-set-2/
One of a good example of Strategy pattern from JDK itself is a Collections.sort() method and Comparator interface, which is a strategy interface and defines a strategy for comparing objects. Because of this pattern, we don't need to modify sort() method (closed for modification) to compare any object, at the same time we can implement Comparator interface to define new comparing strategy (open for extension).
Facade Design Pattern
So, As the name suggests, it means the face of the building. The people walking past the road can only see this glass face of the building. They do not know anything about it, the wiring, the pipes and other complexities. It hides all the complexities of the building and displays a friendly face.
Example : JDBC getConnection();
https://www.geeksforgeeks.org/facade-design-pattern-introduction/
Chain of Responsibility Design Pattern
Chain of Responsibility allows a number of classes to attempt to handle a request, independently of any other object along the chain. Once the request is handled, it completes it’s journey through the chain.
javax.servlet.Filter#doFilter()
The doFilter method of the Filter is called by the container each time a request/response pair is passed through the chain due to a client request for a resource at the end of the chain. The FilterChain passed in to this method allows the Filter to pass on the request and response to the next entity in the chain.
java.util.logging.Logger#log
If the logger is currently enabled for the given message level then the given message is forwarded to all the registered output Handler objects.
Adapter Design Pattern in Java
Adapter design pattern is one of the structural design pattern and its used so that two unrelated interfaces can work together. The object that joins these unrelated interface is called an Adapter.
BufferedReader br = new BufferedReader(new InputStreamReader(System.in));
System.out.print("Enter String");
String s = br.readLine();
System.out.print("Enter input: " + s);
Now observe above code carefully.
1) System.in is static instance of InputStream declared as:
public final static InputStream in = nullInputStream();
This input stream reads the data from console in bytes stream.
2) BufferedReader as java docs define, reads a character stream.
//Reads text from a character-input stream, buffering characters so as to
//provide for the efficient reading of characters, arrays, and lines.
public class BufferedReader extends Reader{..}
Now here is the problem. System.in provides byte stream where BufferedReader expects character stream. How they will work together?
This is the ideal situation to put a adapter in between two incompatible interfaces. InputStreamReader does exactly this thing and works adapter between System.in and BufferedReader.
/** An InputStreamReader is a bridge from byte streams to character streams:
* It reads bytes and decodes them into characters using a specified charset.
* The charset that it uses may be specified by name or may be given explicitly,
* or the platform's default charset may be accepted.
*/
public class InputStreamReader extends Reader {...}
1) java.util.Arrays#asList()
This method accepts multiple strings and return a list of input strings. Though it’s very basic usage, but it’s what a adapter does, right?
2) java.io.OutputStreamWriter(OutputStream)
It’s similar to above usecase we discussed in this post:
Writer writer = new OutputStreamWriter(new FileOutputStream("c:\\data\\output.txt"));
writer.write("Hello World");
3) javax.xml.bind.annotation.adapters.XmlAdapter#marshal() and #unmarshal()
Adapts a Java type for custom marshaling. Convert a bound type to a value type.
No comments:
Post a Comment