What Is Spring Cloud?
Spring Cloud, in microservices, is a system that provides integration with external systems. It is a short-lived framework that builds an application, fast. Being associated with the finite amount of data processing, it plays a very important role in microservice architectures.
For typical use cases, Spring Cloud provides the out of the box experiences and a sets of extensive features mentioned below:
Versioned and distributed configuration.
Discovery of service registration.
Service to service calls.
Routing.
Circuit breakers and load balancing.
Cluster state and leadership election.
Global locks and distributed messaging.
Role of Actuator in Spring Boot
It is one of the most important features, which helps you to access the current state of an application that is running in a production environment. There are multiple metrics which can be used to check the current state. They also provide endpoints for RESTful web services which can be simply used to check the different metrics.
What Is Semantic Monitoring?
It combines monitoring of the entire application along with automated tests. The primary benefit of Semantic Monitoring is to find out the factors which are more profitable to your business.
Semantic monitoring along with service layer monitoring approaches monitoring of microservices from a business point of view. Once an issue is detected, they allow faster isolation and bug triaging, thereby reducing the main time required to repair. It triages the service layer and transaction layer to figure out the transactions affected by availability or poor performance.
How Can You Set Up Service Discovery?
There are multiple ways to set up service discovery. I’ll choose the one that I think to be most efficient, Eureka by Netflix. It is a hassle free procedure that does not weigh much on the application. Plus, it supports numerous types of web applications.
Eureka configuration involves two steps – client configuration and server configuration.
Client configuration can be done easily by using the property files. In the clas spath, Eureka searches for a eureka-client.properties file. It also searches for overrides caused by the environment in property files which are environment specific.
For server configuration, you have to configure the client first. Once that is done, the server fires up a client which is used to find other servers. The Eureka server, by default, uses the Client configuration to find the peer server.
Why Would You Opt for Microservices Architecture?
This is a very common microservices interview question which you should be ready for! There are plenty of pros that are offered by a microservices architecture. Here are a few of them:
Microservices can adapt easily to other frameworks or technologies.
Failure of a single process does not affect the entire system.
Provides support to big enterprises as well as small teams.
Can be deployed independently and in relatively less time.
Define Domain Driven Design
The main focus is on the core domain logic. Complex designs are detected based on the domain’s model. This involves regular collaboration with domain experts to resolve issues related to the domain and improve the model of the application. While answering this microservices interview question, you will also need to mention the core fundamentals of DDD. They are:
DDD focuses mostly on domain logic and the domain itself.
Complex designs are completely based on the domain’s model.
To improve the design of the model and fix any emerging issues, DDD constantly works in collaboration with domain experts.
What Are Coupling and Cohesion?
Coupling can be considered to be the measurement of strength between the dependencies of a component. A good microservices application design always consists of low coupling and high cohesion.
Interviewers will often ask about cohesion. It is also another measurement unit. More like a degree to which the elements inside a module remain bonded together.
It is imperative to keep in mind that an important key to designing microservices is a composition of low coupling along with high cohesion. When loosely coupled, a service knows very little about other services. This keeps the services intact. In high cohesion, it becomes possible to keep all the related logic in a service. Otherwise, the services will try to communicate with each other, impacting the overall performance.
What Is OAuth?
Open Authorization Protocol, otherwise known as OAuth, helps to access client applications using third-party protocols like Facebook, GitHub, etc., via HTTP. You can also share resources between different sites without the requirement of credentials.
OAuth allows the account information of the end user to be used by a third-party like Facebook while keeping it secure (without using or exposing the user’s password). It acts more like an intermediary on the user’s behalf while providing a token to the server for accessing the required information.
Why Do We Need Containers for Microservices?
To manage a microservice-based application, containers are the easiest alternative. It helps the user to individually deploy and develop. You can also use Docker to encapsulate microservices in the image of a container. Without any additional dependencies or effort, microservices can use these elements.
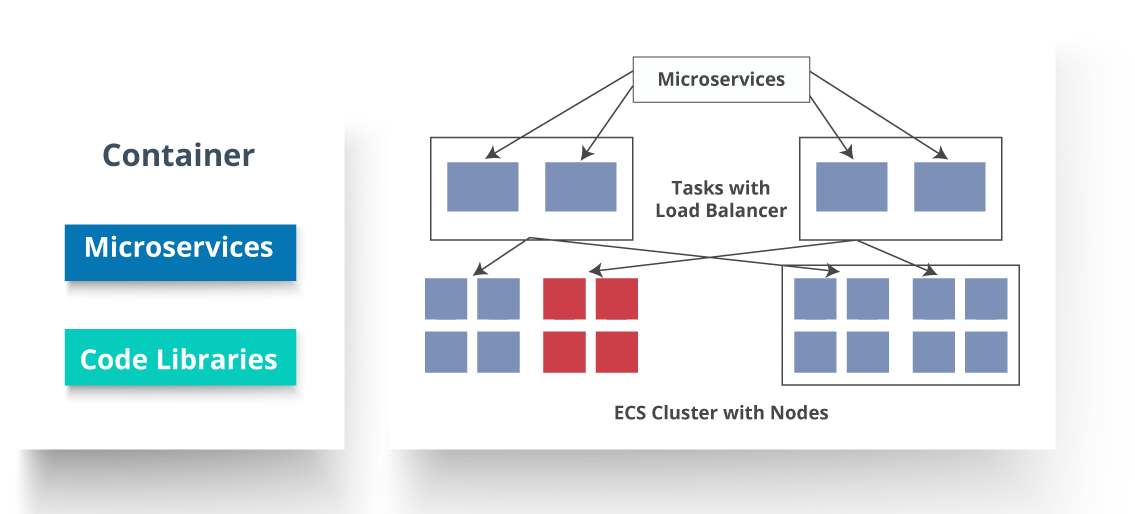
What Are the Ways to Access RESTful Microservices?
Another one of the frequently asked microservices interview questions is how to access RESTful microservices? You can do that via two methods:
Using a REST template that is load balanced.
Using multiple microservices.
What Are Some Major Roadblocks for Microservices Testing?
https://www.lambdatest.com/blog/testing-challenges-related-to-microservice-architecture/
Common Mistakes Made While Transitioning to Microservices
Not only on development, but mistakes also often occur on the process side. And any experienced interviewer will have this in the queue for microservices interview questions. Some of the common mistakes are:
Often the developer fails to outline the current challenges.
Rewriting the programs that are already existing.
Responsibilities, timeline, and boundaries not clearly defined.
Failing to implement and figure out the scope of automation from the very beginning.
What Are the Fundamentals of Microservices Design?
This is probably one of the most frequently asked microservices interview questions. Here is what you need to keep in mind while answering to it:
Define a scope.
Combine loose coupling with high cohesion.
Create a unique service which will act as an identifying source, much like a unique key in a database table.
Creating the correct API and taking special care during integration.
Restrict access to data and limit it to the required level.
Maintain a smooth flow between requests and response.
Automate most processes to reduce time complexity.
Keep the number of tables to a minimum level to reduce space complexity.
Monitor the architecture constantly and fix any flaw when detected.
Data stores should be separated for each microservice.
For each microservice, there should be an isolated build.
Deploy microservices into containers.
Servers should be treated as stateless.
Where Do We Use WebMVC Test Annotation?
WebMvcTest is used for unit testing Spring MVC applications. As the name suggests, it focuses entirely on Spring MVC components. For example,
@WebMvcTest(value = ToTestController.class, secure = false):
Here, the objective is to only launch ToTestController. Until the unit test has been executed, other mappings and controllers will not be launched.
What Do You Mean by Bounded Context?
A central pattern is usually seen in domain driven design. Bounded context is the main focus of the strategic design section of DDD. It is all about dealing with large teams and models. DDD works with large models by disintegrating them into multiple bounded contexts. While it does that, it also explains the relationship between them explicitly.
What Are the Different Types of Two-Factor Authentication?
There are three types of credentials required for performing two-factor authentication.
A thing that you know – like password or pin or screen lock pattern.
A physical credential that you have – like OTP or phone or an ATM card, in other words, any kind of credential that you have in an external or third-party device.
Your physical identity – like voice authentication or biometric security, like a fingerprint or eye scanner.
What is Conway’s law?
“Any organization that designs a system (defined broadly) will produce a design whose structure is a copy of the organization’s communication structure.” – Mel Conway
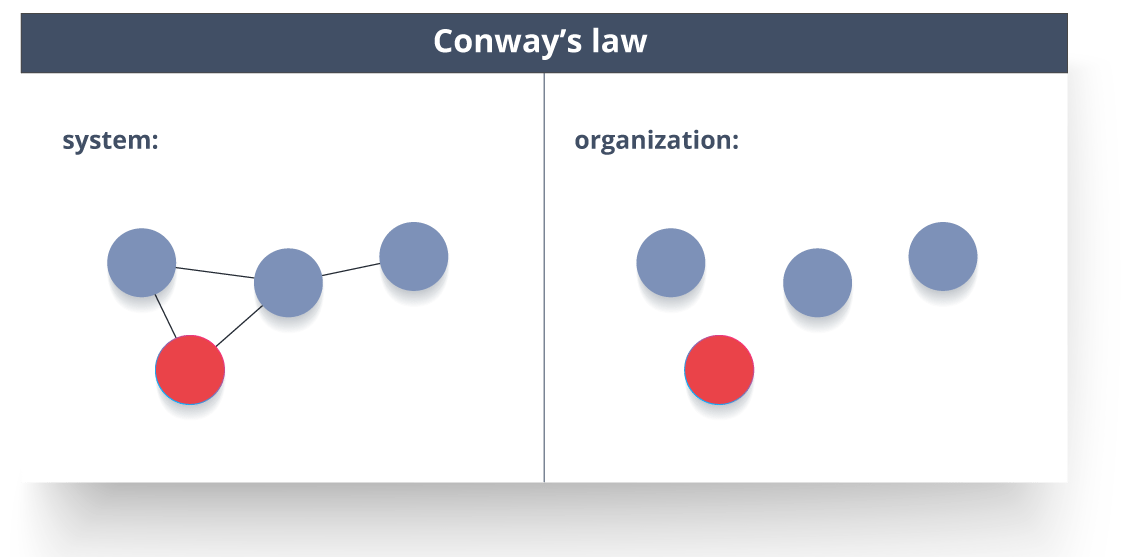
This law basically tries to convey the fact that, in order for a software module to function, the complete team should communicate well. Therefore the structure of a system reflects the social boundaries of the organization(s) that produced it.
What Is Idempotence and How Is it Used?
Idempotence refers to a scenario where you perform a task repetitively but the end result remains constant or similar.
Idempotence is mostly used as a data source or a remote service in a way that when it receives more than one set of instructions, it processes only one set of instructions.
Best Practices to Design MS :
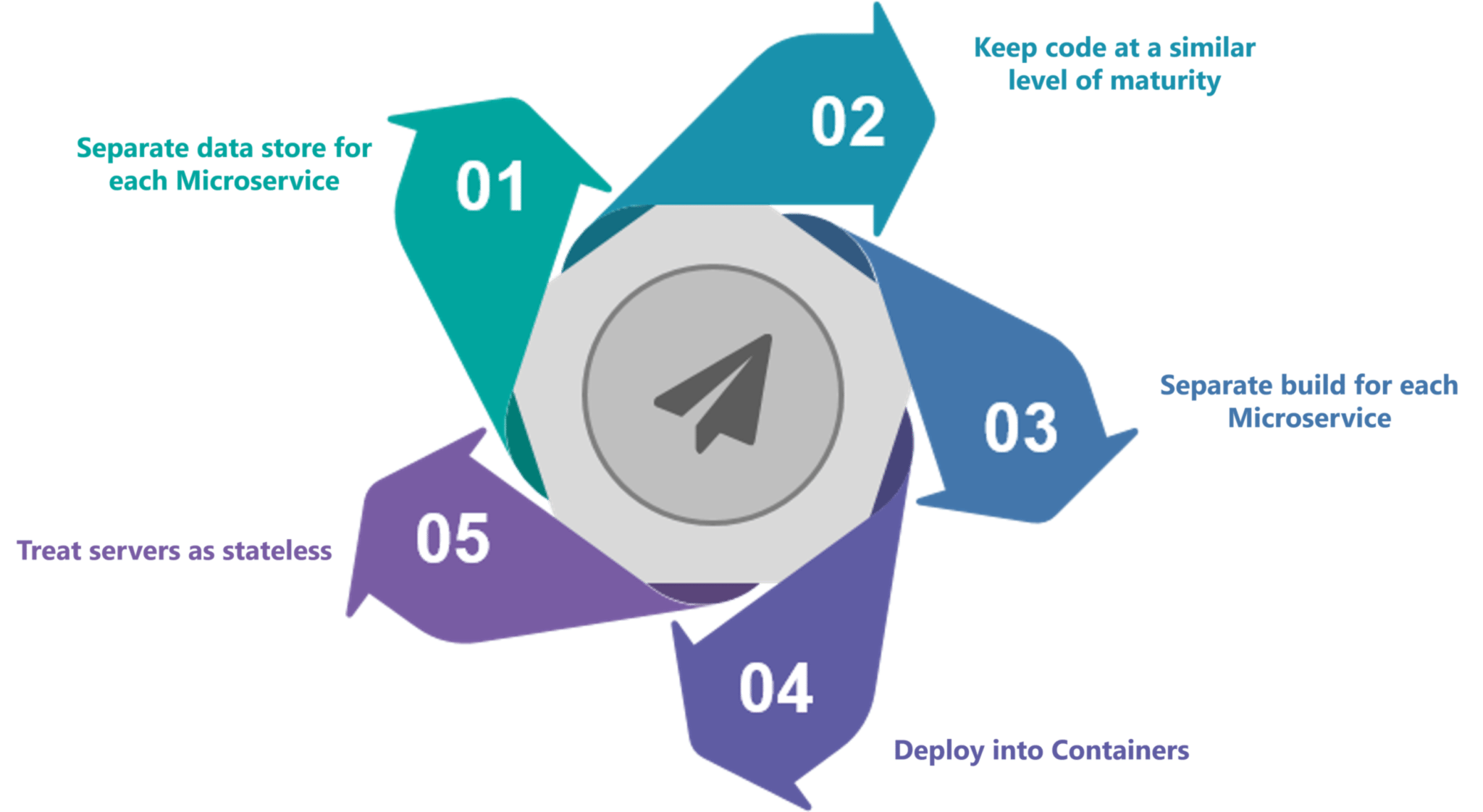
Spring Cloud, in microservices, is a system that provides integration with external systems. It is a short-lived framework that builds an application, fast. Being associated with the finite amount of data processing, it plays a very important role in microservice architectures.
For typical use cases, Spring Cloud provides the out of the box experiences and a sets of extensive features mentioned below:
Versioned and distributed configuration.
Discovery of service registration.
Service to service calls.
Routing.
Circuit breakers and load balancing.
Cluster state and leadership election.
Global locks and distributed messaging.
Role of Actuator in Spring Boot
It is one of the most important features, which helps you to access the current state of an application that is running in a production environment. There are multiple metrics which can be used to check the current state. They also provide endpoints for RESTful web services which can be simply used to check the different metrics.
What Is Semantic Monitoring?
It combines monitoring of the entire application along with automated tests. The primary benefit of Semantic Monitoring is to find out the factors which are more profitable to your business.
Semantic monitoring along with service layer monitoring approaches monitoring of microservices from a business point of view. Once an issue is detected, they allow faster isolation and bug triaging, thereby reducing the main time required to repair. It triages the service layer and transaction layer to figure out the transactions affected by availability or poor performance.
How Can You Set Up Service Discovery?
There are multiple ways to set up service discovery. I’ll choose the one that I think to be most efficient, Eureka by Netflix. It is a hassle free procedure that does not weigh much on the application. Plus, it supports numerous types of web applications.
Eureka configuration involves two steps – client configuration and server configuration.
Client configuration can be done easily by using the property files. In the clas spath, Eureka searches for a eureka-client.properties file. It also searches for overrides caused by the environment in property files which are environment specific.
For server configuration, you have to configure the client first. Once that is done, the server fires up a client which is used to find other servers. The Eureka server, by default, uses the Client configuration to find the peer server.
Why Would You Opt for Microservices Architecture?
This is a very common microservices interview question which you should be ready for! There are plenty of pros that are offered by a microservices architecture. Here are a few of them:
Microservices can adapt easily to other frameworks or technologies.
Failure of a single process does not affect the entire system.
Provides support to big enterprises as well as small teams.
Can be deployed independently and in relatively less time.
Define Domain Driven Design
The main focus is on the core domain logic. Complex designs are detected based on the domain’s model. This involves regular collaboration with domain experts to resolve issues related to the domain and improve the model of the application. While answering this microservices interview question, you will also need to mention the core fundamentals of DDD. They are:
DDD focuses mostly on domain logic and the domain itself.
Complex designs are completely based on the domain’s model.
To improve the design of the model and fix any emerging issues, DDD constantly works in collaboration with domain experts.
What Are Coupling and Cohesion?
Coupling can be considered to be the measurement of strength between the dependencies of a component. A good microservices application design always consists of low coupling and high cohesion.
Interviewers will often ask about cohesion. It is also another measurement unit. More like a degree to which the elements inside a module remain bonded together.
It is imperative to keep in mind that an important key to designing microservices is a composition of low coupling along with high cohesion. When loosely coupled, a service knows very little about other services. This keeps the services intact. In high cohesion, it becomes possible to keep all the related logic in a service. Otherwise, the services will try to communicate with each other, impacting the overall performance.
What Is OAuth?
Open Authorization Protocol, otherwise known as OAuth, helps to access client applications using third-party protocols like Facebook, GitHub, etc., via HTTP. You can also share resources between different sites without the requirement of credentials.
OAuth allows the account information of the end user to be used by a third-party like Facebook while keeping it secure (without using or exposing the user’s password). It acts more like an intermediary on the user’s behalf while providing a token to the server for accessing the required information.
Why Do We Need Containers for Microservices?
To manage a microservice-based application, containers are the easiest alternative. It helps the user to individually deploy and develop. You can also use Docker to encapsulate microservices in the image of a container. Without any additional dependencies or effort, microservices can use these elements.
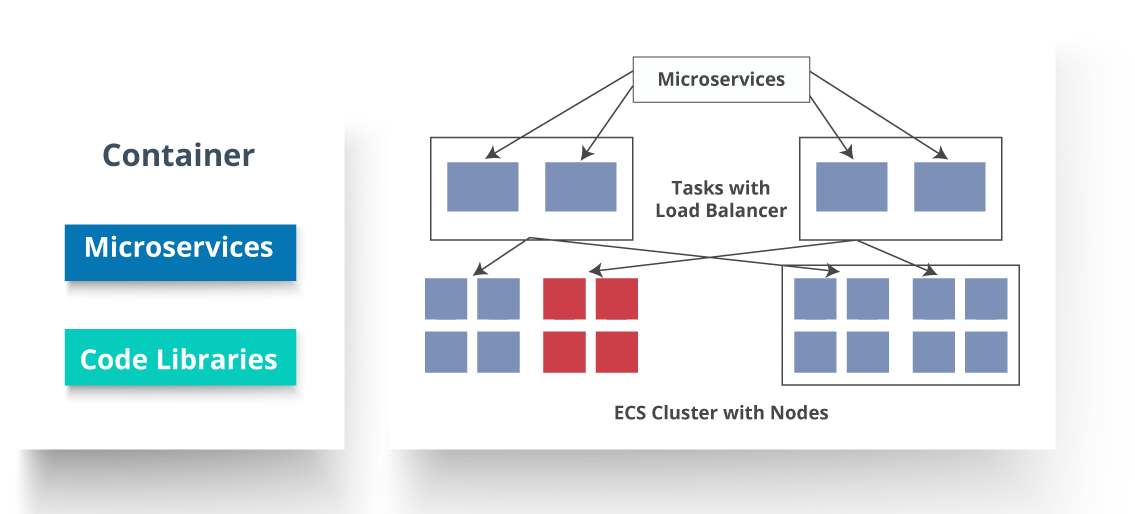
What Are the Ways to Access RESTful Microservices?
Another one of the frequently asked microservices interview questions is how to access RESTful microservices? You can do that via two methods:
Using a REST template that is load balanced.
Using multiple microservices.
What Are Some Major Roadblocks for Microservices Testing?
https://www.lambdatest.com/blog/testing-challenges-related-to-microservice-architecture/
Common Mistakes Made While Transitioning to Microservices
Not only on development, but mistakes also often occur on the process side. And any experienced interviewer will have this in the queue for microservices interview questions. Some of the common mistakes are:
Often the developer fails to outline the current challenges.
Rewriting the programs that are already existing.
Responsibilities, timeline, and boundaries not clearly defined.
Failing to implement and figure out the scope of automation from the very beginning.
What Are the Fundamentals of Microservices Design?
This is probably one of the most frequently asked microservices interview questions. Here is what you need to keep in mind while answering to it:
Define a scope.
Combine loose coupling with high cohesion.
Create a unique service which will act as an identifying source, much like a unique key in a database table.
Creating the correct API and taking special care during integration.
Restrict access to data and limit it to the required level.
Maintain a smooth flow between requests and response.
Automate most processes to reduce time complexity.
Keep the number of tables to a minimum level to reduce space complexity.
Monitor the architecture constantly and fix any flaw when detected.
Data stores should be separated for each microservice.
For each microservice, there should be an isolated build.
Deploy microservices into containers.
Servers should be treated as stateless.
Where Do We Use WebMVC Test Annotation?
WebMvcTest is used for unit testing Spring MVC applications. As the name suggests, it focuses entirely on Spring MVC components. For example,
@WebMvcTest(value = ToTestController.class, secure = false):
Here, the objective is to only launch ToTestController. Until the unit test has been executed, other mappings and controllers will not be launched.
What Do You Mean by Bounded Context?
A central pattern is usually seen in domain driven design. Bounded context is the main focus of the strategic design section of DDD. It is all about dealing with large teams and models. DDD works with large models by disintegrating them into multiple bounded contexts. While it does that, it also explains the relationship between them explicitly.
What Are the Different Types of Two-Factor Authentication?
There are three types of credentials required for performing two-factor authentication.
A thing that you know – like password or pin or screen lock pattern.
A physical credential that you have – like OTP or phone or an ATM card, in other words, any kind of credential that you have in an external or third-party device.
Your physical identity – like voice authentication or biometric security, like a fingerprint or eye scanner.
What is Conway’s law?
“Any organization that designs a system (defined broadly) will produce a design whose structure is a copy of the organization’s communication structure.” – Mel Conway
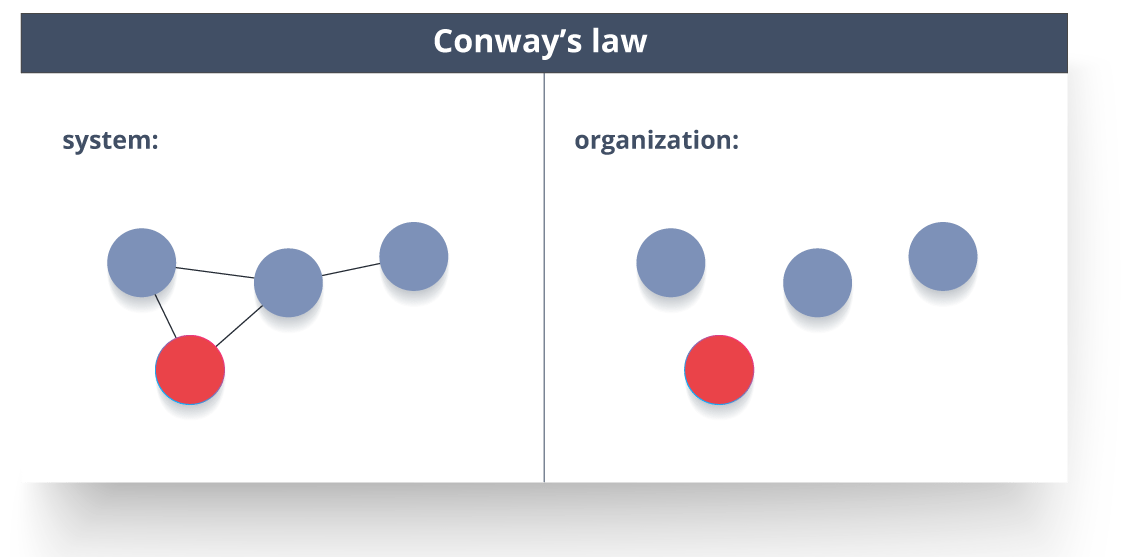
This law basically tries to convey the fact that, in order for a software module to function, the complete team should communicate well. Therefore the structure of a system reflects the social boundaries of the organization(s) that produced it.
What Is Idempotence and How Is it Used?
Idempotence refers to a scenario where you perform a task repetitively but the end result remains constant or similar.
Idempotence is mostly used as a data source or a remote service in a way that when it receives more than one set of instructions, it processes only one set of instructions.
List down the advantages of Microservices Architecture.
Advantage | Description |
Independent Development | All microservices can be easily developed based on their individual functionality |
Independent Deployment | Based on their services, they can be individually deployed in any application |
Fault Isolation | Even if one service of the application does not work, the system still continues to function |
Mixed Technology Stack | Different languages and technologies can be used to build different services of the same application |
Granular Scaling | Individual components can scale as per need, there is no need to scale all components together |
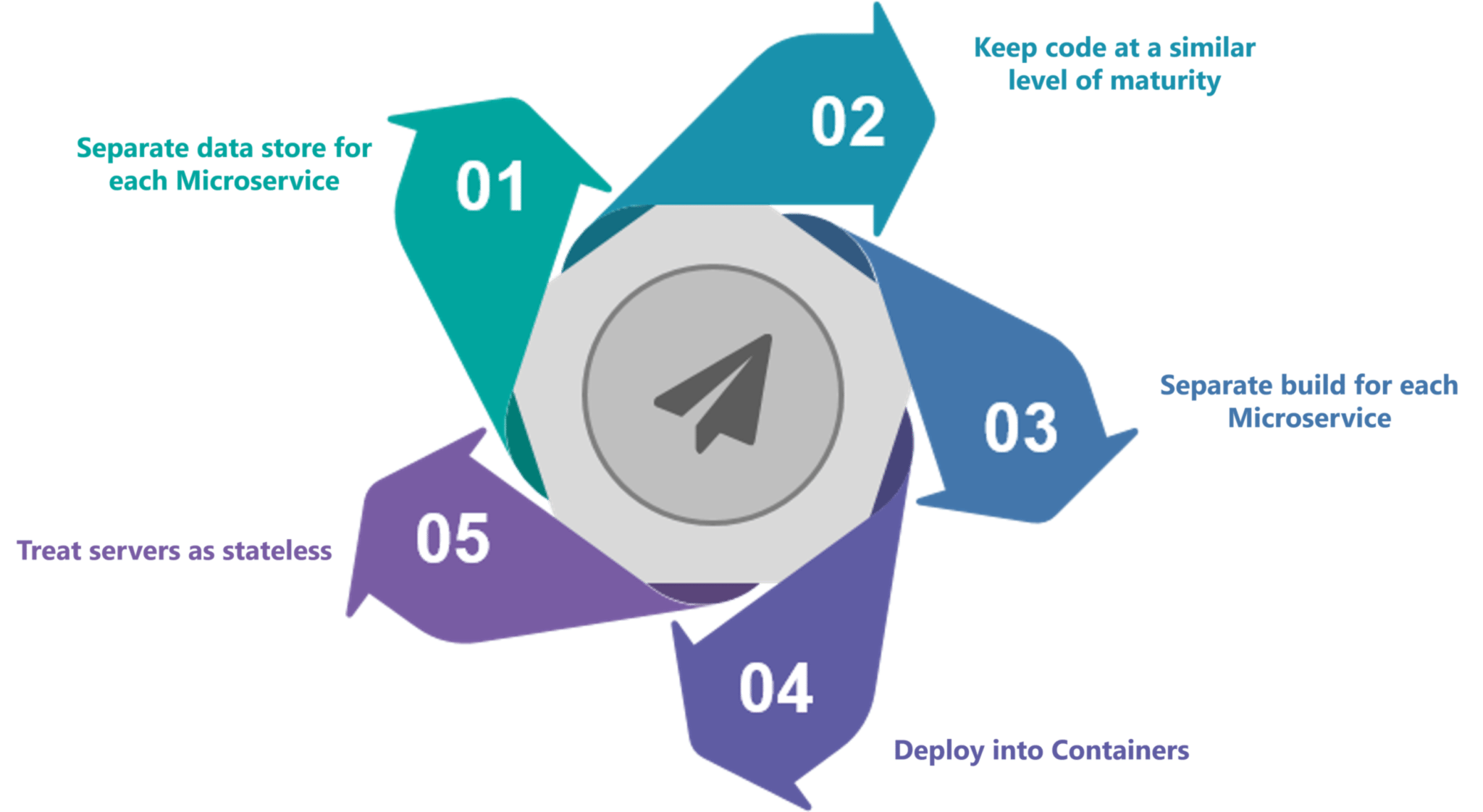
What are the pros and cons of Microservice Architecture?
What is the difference between Monolithic, SOA and Microservices Architecture?Pros of Microservice Architecture | Cons of Microservice Architecture |
Freedom to use different technologies | Increases troubleshooting challenges |
Each microservices focuses on single capability | Increases delay due to remote calls |
Supports individual deployable units | Increased efforts for configuration and other operations |
Allow frequent software releases | Difficult to maintain transaction safety |
Ensures security of each service | Tough to track data across various boundaries |
Mulitple services are parallelly developed and deployed | Difficult to code between services |
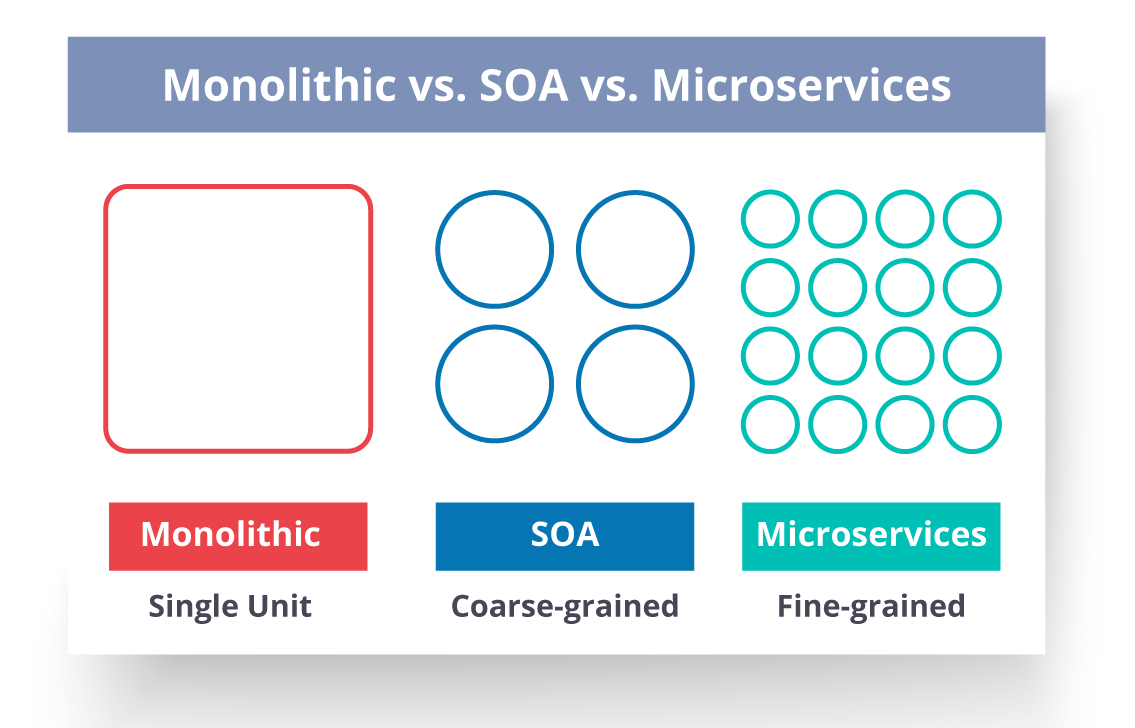
Monolithic Architecture is similar to a big container wherein all the software components of an application are assembled together and tightly packaged.
A Service-Oriented Architecture is a collection of services which communicate with each other. The communication can involve either simple data passing or it could involve two or more services coordinating some activity.
Microservice Architecture is an architectural style that structures an application as a collection of small autonomous services, modeled around a business domain.
What are the challenges you face while working Microservice Architectures?
Developing a number of smaller microservices sounds easy, but the challenges often faced while developing them are as follows.
Automate the Components: Difficult to automate because there are a number of smaller components. So for each component, we have to follow the stages of Build, Deploy and, Monitor.
Perceptibility: Maintaining a large number of components together becomes difficult to deploy, maintain, monitor and identify problems. It requires great perceptibility around all the components.
Configuration Management: Maintaining the configurations for the components across the various environments becomes tough sometimes.
Debugging: Difficult to find out each and every service for an error. It is essential to maintain centralized logging and dashboards to debug problems.
What are the key differences between SOA and Microservices Architecture?
The key differences between SOA and microservices are as follows:
SOA | Microservices |
Follows “share-as-much-as-possible” architecture approach | Follows “share-as-little-as-possible” architecture approach |
Importance is on business functionality reuse | Importance is on the concept of “bounded context” |
They have common governance and standards | They focus on people collaboration and freedom of other options |
Uses Enterprise Service bus (ESB) for communication | Simple messaging system |
They support multiple message protocols | They use lightweight protocols such as HTTP/REST etc. |
Multi-threaded with more overheads to handle I/O | Single-threaded usually with the use of Event Loop features for non-locking I/O handling |
Maximizes application service reusability | Focuses on decoupling |
Traditional Relational Databases are more often used | Modern Relational Databases are more often used |
A systematic change requires modifying the monolith | A systematic change is to create a new service |
DevOps / Continuous Delivery is becoming popular, but not yet mainstream | Strong focus on DevOps / Continuous Delivery |
What do you understand by Distributed Transaction?
Distributed Transaction is any situation where a single event results in the mutation of two or more separate sources of data which cannot be committed atomically. In the world of microservices, it becomes even more complex as each service is a unit of work and most of the time multiple services have to work together to make a business successful.
Idempotence is used at the remote service, or data source so that, when it receives the instruction more than once, it only processes the instruction once.
What do you understand by Contract Testing?
According to Martin Flower, contract test is a test at the boundary of an external service which verifies that it meets the contract expected by a consuming service.
Also, contract testing does not test the behavior of the service in depth. Rather, it tests that the inputs & outputs of service calls contain required attributes and the response latency, throughput is within allowed limits.
What is the difference between Mock or Stub?
Stub
A dummy object that helps in running the test.
Provides fixed behavior under certain conditions which can be hard-coded.
Any other behavior of the stub is never tested.
For example, for an empty stack, you can create a stub that just returns true for empty() method. So, this does not care whether there is an element in the stack or not.
Mock
A dummy object in which certain properties are set initially.
The behavior of this object depends on the set properties.
The object’s behavior can also be tested.
For example, for a Customer object, you can mock it by setting name and age. You can set age as 12 and then test for isAdult() method that will return true for age greater than 18. So, your Mock Customer object works for the specified condition.
What do you mean by Continuous Integration (CI)?
Continuous Integration (CI) is the process of automating the build and testing of code every time a team member commits changes to version control. This encourages developers to share code and unit tests by merging the changes into a shared version control repository after every small task completion.
Can we create State Machines out of Microservices?
As we know that each Microservice owning its own database is an independently deployable program unit, this, in turn, lets us create a State Machine out of it. So, we can specify different states and events for a particular microservice.
For Example, we can define an Order microservice. An Order can have different states. The transitions of Order states can be independent events in the Order microservice.
What are Reactive Extensions in Microservices?
Reactive Extensions also are known as Rx. It is a design approach in which we collect results by calling multiple services and then compile a combined response. These calls can be synchronous or asynchronous, blocking or non-blocking. Rx is a very popular tool in distributed systems which works opposite to legacy flows.
How would you test MS based Architecture?
One should have unit and integration tests where all the functionality of a microservice can be tested. One should also have component based testing.
One should have contract tests to assert that the expectations by the client is not breaking. End-to-end test for the microservices, however, should only test the critical flows as these can be time-consuming. The tests can be from two sides, consumer-driven contract test and consumer-side contract test.
You can also leverage Command Query Responsibility Segregation to query multiple databases and get a combined view of persisted data.
What is Service Discovery? How is it helpful.
In a cloud environment where docker images are dynamically deployed on any machine or IP + Port combination, it becomes difficult for dependent services to update at runtime. Service discovery is created due to that purpose only.
Service discovery is one of the services running under microservices architecture, which registers entries of all of the services running under the service mesh. All of the actions are available through the REST API. So whenever the services are up and running, the individual services registers themselves to service discovery service and service discovery services maintains heartbeat to make sure that those services are alive. That also serves the purpose of monitoring services as well. Service discovery also helps in distributing requests across services deployed in a fair manner.
Client Side & Server Side Discovery
Instead of clients directly connecting to load balancer, in this architectural pattern the client connects to the service registry and tries to fetch data or services from it.
Once it gets all data, it does load balancing on its own and directly reaches out to the services it needs to talk to.
This can have a benefit where there are multiple proxy layers and delays are happening due to the multilayer communication.
In server-side discovery, the proxy layer or API Gateway later tries to connect to the service registry and makes a call to appropriate service afterward. Over here client connects to that proxy layer or API Gateway layer.
Explain how can you scale a microservice based system?
Assuming that the majority of providers using microservices architecture,
A. One can scale the system by increasing the number of instances of service by bringing up more containers.
B. One can also apply to cache at microservice layer which can be easy to manage as an invalidation of the cache can be done very easily as the microservice will be the single source of truth.
C. Caching can also be introduced at the API Gateway layer where one can define caching rules like when to invalidate the cache.
D. One can also shut down some containers when the requirement is less. That is, scale down.
Define Microservices Architecture?
Microservices Architecture is a style of developing a scalable, distributed & highly automated system made up of many small autonomous services. It is not a technology but a new trend evolved out of SOA.
There is no single definition that fully describes the term "microservices". Some of the famous authors have tried to define it in the following way:
Microservices are small, autonomous services that work together.
Loosely coupled service-oriented architecture with bounded contexts.
Microservice architecture is a natural consequence of applying the single responsibility principle at the architectural level.
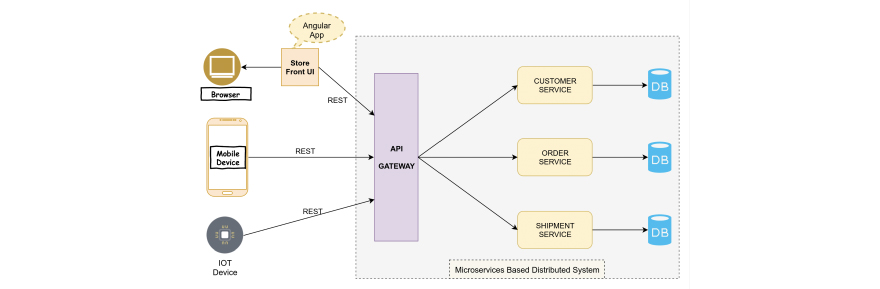
Difference between Microservices and SOA
Microservices are a continuation to SOA.
SOA started gaining ground due to its distributed architecture approach and it emerged to combat the problems of large monolithic applications, around 2006.
Both (SOA and Microservices) of these architectures share one common thing that they both are distributed architecture and both allow high scalability. In both, service components are accessed remotely through remote access protocol (RMI, REST, SOAP, AMQP, JMS, etc.). both are modular and loosely coupled by design and offer high scalability. Microservices started gaining buzz in late 2000 after the emergence of lightweight containers, Docker, Orchestration Frameworks (Kubernetes, Mesos). Microservices differ from SOA in a significant manner conceptually -
SOA uses Enterprise Service Bus for communication, while microservices uses REST or some other less elaborate messaging system (AMQP, etc). Also, microservice follow "Smart endpoints and dumb points", which means that when a microservice needs another one as a dependency, it should use it directly without any routing logic/components handling the pipe.
In microservices, service deployment and management should be fully automated, whereas SOA services are often implemented in deployment monoliths.
Generally, microservices are significantly smaller than what SOA tends to be. Here we are not talking about the codebase here because few languages are more verbose than the other ones. We are talking about the scope (problem domain) of the service itself. Microservices generally do one thing in a better way.
Microservices should own their own data while SOA may share a common database. So one Microservices should not allow another Microservices to change/read its data directly.
Classic SOA is more platform-driven, while we have a lot of technology independence in case of microservices. Each microservice can have its own technology stack based on its own functional requirements. So microservices offers more choices in all dimensions.
Microservices make an as little assumption as possible on the external environment. A Microservice should manage its own functional domain and data model.
What is Bounded Context?
Bounded Context is a central pattern in Domain-Driven Design. In Bounded Context, everything related to the domain is visible within context internally but opaque to other bounded contexts. DDD deals with large models by dividing them into different Bounded Contexts and being explicit about their interrelationships.
Monolithic Conceptual Model Problem
A single conceptual model for the entire organization is very tricky to deal with. The only benefit of such a unified model is that integration is easy across the whole enterprise, but the drawbacks are many, for example:
At first, it's very hard to build a single model that works for the entire organization.
It's hard for others (teams) to understand it.
It's very difficult to change such a shared model to accommodate the new business requirements. The impact of such a change will be widespread across team boundaries.
Any large enterprise needs a model that is either very large or abstract.
Meaning of a single word may be different in different departments of an organization, so it may be really difficult to come up with a single unified model. Such a model, even if created, will lead to a lot of confusion across the teams.
Characteristics of a microservices architecture
High Cohesion - Small and focussed on doing one thing well. Small does not mean less number of lines of code because few programming languages are more verbose than others, but it means the smallest functional area that a single microservices caters to.
Loose Coupling - Autonomous - the ability to deploy different services independently, and reliability, due to the ability for a service to run even if another service is down.
Bounded Context - A Microservice serves a bounded context in a domain. It communicates with the rest of the domain by using an interface for that Bounded context.
Organisation around business capabilities instead of around technology.
Continuous Delivery and Infrastructure automation.
Versioning for backward compatibility. Even multiple versions of same microservices can exist in a production environment.
Fault Tolerance - if one service fails, it will not affect the rest of the system. For example, if a microservices serving the comments and reviews for e-commerce fails, the rest of the website should run fine.
Decentralized data management with each service owning its database rather than a single shared database. Every microservice has the freedom to choose the right type of database appropriate for its business use-case (for example, RDBMS for Order Management, NoSql for catalogue management for an e-commerce website)
Eventual Consistency - event-driven asynchronous updates.
Security - Every microservice should have the capability to protect its own resources from unauthorized access. This is achieved using stateless security mechanisms like JSON Web Token (JWT pronounced as jot) with OAuth2.
What are the benefits of using microservices architecture?
Embracing microservices architecture brings many benefits compared to using monolith architecture in your application, including:
Autonomous Deployments
The decentralized teams working on individual microservices are mostly independent of each other, so changing a service does not require coordination with other teams. This can lead to significantly faster release cycles. It is very hard to achieve the same thing in a realistic monolithic application where a small change may require regression of the entire system.
Culture Shift
Microservices style of system architecture emphasizes on the culture of freedom, single responsibility, autonomy of teams, faster release iterations and technology diversification.
Technology Diversification
Unlike in monolithic applications, microservices are not bound to one technology stack (Java, .Net, Go, Erlang, Python, etc). Each team is free to choose a technology stack that is best suited for its requirements. For example, we are free to choose Java for a microservice, c++ for others and Go for another one.
DevOps Culture
The term comes from an abbreviated compound of "development" and "operations". It is a culture that emphasizes effective communication and collaboration between product management, software development, and operations team. DevOps culture, if implemented correctly can lead to shorter development cycles and thus faster time to market.
What is polyglot persistence? Can this idea be used in monolithic applications as well?
Polyglot persistence is all about using different databases for different business needs within a single distributed system. We already have different database products in the market each for a specific business need, for example:
RDBMS
Relational databases are used for transactional needs (storing financial data, reporting requirements, etc.)
MongoDB
Documented oriented databases are used for documents oriented needs (for e.g. Product Catalog). Documents are schema-free so changes in the schema can be accommodated into the application without much headache.
Cassandra/Amazon DynamoDB
Key-value pair based database (User activity tracking, Analytics, etc.). DynamoDB can store documents as well as key-value pairs.
Redis
In memory distributed database (user session tracking), its mostly used as a distributed cache among multiple microservices.
Neo4j
Graph DB (social connections, recommendations, etc)
Benefits of Polyglot Persistence are manifold and can be harvested in both monolithic as well as microservices architecture. Any decent-sized product will have a variety of needs which may not be fulfilled by a single kind of database alone. For example, if there are no transactional needs for a particular microservice, then it's way better to use a key-value pair or document-oriented NoSql rather than using a transactional RDBMS database.
References: https://martinfowler.com/bliki/PolyglotPersistence.html
What is the 12 Factor App?
The Twelve-Factor App is a recent methodology (and/or a manifesto) for writing web applications which run as a service.
Codebase
One codebase, multiple deploys. This means that we should only have one codebase for different versions of a microservices. Branches are ok, but different repositories are not.
Dependencies
Explicitly declare and isolate dependencies. The manifesto advises against relying on software or libraries on the host machine. Every dependency should be put into pom.xml or build.gradle file.
Config
Store config in the environment. Do never commit your environment-specific configuration (most importantly: password) in the source code repo. Spring Cloud Config provides server and client-side support for externalized configuration in a distributed system. Using Spring Cloud Config Server you have a central place to manage external properties for applications across all environments.
Backing services
Treat backing services as attached resources. A microservice should treat external services equally, regardless of whether you manage them or some other team. For example, never hard code the absolute url for dependent service in your application code, even if the dependent microservice is developed by your own team. For example, instead of hard coding url for another service in your RestTemplate, use Ribbon (with or without Eureka) to define the url:
Release & Run
Strictly separate build and run stages. In other words, you should be able to build or compile the code, then combine that with specific configuration information to create a specific release, then deliberately run that release. It should be impossible to make code changes at runtime, for e.g. changing the class files in tomcat directly. There should always be a unique id for each version of release, mostly a timestamp. Release information should be immutable, any changes should lead to a new release.
Processes
Execute the app as one or more stateless processes. This means that our microservices should be stateless in nature, and should not rely on any state being present in memory or in the filesystem. Indeed the state does not belong in the code. So no sticky sessions, no in-memory cache, no local filesystem storage, etc. Distributed cache like memcache, ehcache or Redis should be used instead
Port Binding
Export services via port binding. This is about having your application as standalone, instead of relying on a running instance of an application server, where you deploy. Spring boot provides a mechanism to create a self-executable uber jar that contains all dependencies and embedded servlet container (jetty or tomcat).
Concurrency
Scale-out via the process model. In the twelve-factor app, processes are a first-class citizen. This does not exclude individual processes from handling their own internal multiplexing, via threads inside the runtime VM, or the async/evented model found in tools such as EventMachine, Twisted, or Node.js. But an individual VM can only grow so large (vertical scale), so the application must also be able to span multiple processes running on multiple physical machines. Twelve-factor app processes should never write PID files, rather it should rely on operating system process manager such as systemd - a distributed process manager on a cloud platform.
Disposability
The twelve-factor app’s processes are disposable, meaning they can be started or stopped at a moment’s notice. This facilitates fast elastic scaling, rapid deployment of code or config changes, and robustness of production deploys. Processes should strive to minimize startup time. Ideally, a process takes a few seconds from the time the launch command is executed until the process is up and ready to receive requests or jobs. Short startup time provides more agility for the release process and scaling up; and it aids robustness because the process manager can more easily move processes to new physical machines when warranted.
Dev/Prod parity
Keep development, staging, and production as similar as possible. Your development environment should almost identical to a production one (for example, to avoid some “works on my machine” issues). That doesn’t mean your OS has to be the OS running in production, though. Docker can be used for creating logical separation for your microservices.
Logs
Treat logs as event streams, sending all logs only to stdout. Most Java Developers would not agree to this advice, though.
Admin processes
Run admin/management tasks as one-off processes. For example, a database migration should be run using a separate process altogether.
What are the challenges in microservices?
DevOps is a must, because of the explosion of a number of processes in a production system. How to start and stop the fleet of services?
The complexity of distributed computing such as “network latency, fault tolerance, message serialization, unreliable networks, handling asynchronous o/p, varying loads within our application tiers, distributed transactions, etc.”
How to make configuration changes across the large fleet of services with minimal effort?
How to deploy multiple versions of single microservice and route calls appropriately?
How to disconnect a microservice from ecosystem when it starts to crash unexpectedly?
How to isolate a failed microservice and avoid cascading failures in the entire ecosystem?
How to discover services in an elastic manner considering that services may be going UP or DOWN at any point in time?
How to aggregate logs/metrics across the services? How to identify different steps of a single client request spread across a span of microservices?
How to convert the large application into microservices architecture?
Microservices should be autonomous and divided based on business capabilities. Each software component should have single well-defined responsibility (a.k.a Single Responsibility Principle) and the principle of Bounded Context (as defined by Domain Driven Design) should be used to create highly cohesive software components.
For example, an e-commerce site can be partitioned into following microservices based on its business capabilities:
Product catalogue
Responsible for product information, searching products, filtering products & products facets.
Inventory
Responsible for managing inventory of products (stock/quantity and facet).
Product review and feedback
Collecting feedback from users about the products.
Orders
Responsible for creating and managing orders.
Payments
Process payments both online and offline (Cash On Delivery).
Shipments
Manage and track shipments against orders.
Demand generation
Market products to relevant users.
User Accounts
Manage users and their preferences.
Recommendations
Suggest new products based on the user’s preference or past purchases.
Notifications
Email and SMS notification about orders, payments, and shipments.
The client application (browser, mobile app) will interact with these services via API gateway and render the relevant information to the user.
No comments:
Post a Comment